AGIWiki/Logic syntax
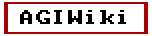
This article describes the logic syntax for the C-like "official" AGI syntax described in the AGI Specs and supported by AGI Studio and WinAGI.
Note: The WinAGI development environment supports an alternate syntax, that is based on Microsoft's Visual Basic language, rather than the C language. At this time, the C-based syntax described in this article is the most widely-supported AGI logic syntax.
Action Commands
Normal action commands are specified by the command name followed by parentheses which contain the arguments, separated by commas. A semicolon is placed after the parentheses. The parentheses are required even if there are no arguments. The arguments given must have the correct prefix for that type of argument as explained later in this document (this is to make sure the programmer does not use a variable, for example, when they think they are using a flag).
assign.v(v50,0);
program.control();
Multiple commands may be placed on the one line:
reset(f6); reset(f7);
Substitutions for the following action commands are available:
increment(v30); v30++;
decrement(v30); v30--;
assignn(v30,4); v30 = 4;
assignv(v30,v32); v30 = v32;
addn(v30,4); v30 = v30 + 4; or v30 += 4;
addv(v30,v32); v30 = v30 + v32; or v30 += v32;
subn(v30,4); v30 = v30 - 4; or v30 -= 4;
subv(v30,v32); v30 = v30 - v32; or v30 -= v32;
mul.n(v30,4); v30 = v30 * 4; or v30 *= 4;
mul.v(v30,v32); v30 = v30 * v32; or v30 *= v32;
div.n(v30,4); v30 = v30 / 4; or v30 /= 4;
div.v(v30,v32); v30 = v30 / v32; or v30 /= v32;
lindirectn(v30,4); *v30 = 4;
lindirectv(v30,v32); *v30 = v32;
rindirect(v30,v32); v30 = *v32;
If structures and test commands
An if structure looks like this:
if (test commands) {
action commands
}
or like this
if (test commands) {
action commands
}
else {
more action commands
}
Carriage returns are not necessary:
if (test commands) { action Commands } else { more action commands }
Test commands are coded like action commands except there is no semicolon. They are separated by &&
or ||
for AND and OR, respectively:
if (isset(f5) &&
greatern(v5,6)) { ......
Again, carriage returns are not necessary within the if statement:
if (lessn(v5,6) && (greatern(v5,2)) { .......
if (isset(f90) && equalv(v32,v34) &&
greatern(v34,20)) { .......
A ! placed in front of a command signifies a NOT.
if (!isset(f7)) {
......
Boolean expressions are not necessarily simplified so they must follow the rules set down by the file format. If test commands are to be ORred together, they must be placed in brackets.
if ((isset(f1) || isset(f2)) {
......
if (isset(f1) && (isset(f2) || isset(f3))) {
......
if (isset(1) || (isset(2) && isset(3))) { is NOT legal
Depending on the compiler, simplification of boolean expressions may be supported, so the above may not apply in all cases (although if these are rules are followed then the logic will work with all compilers).
Substitutions for the following test commands are available:
equaln(v30,4) v30 == 4
equalv(v30,v32) v30 == v32
greatern(v30,4) v30 4
greaterv(v30,v32) v30 v32
lessn(v30,4) v30 4
lessv(v30,v32) v30 v32
!equaln(v30,4) v30 != 4
!equalv(v30,v32) v30 != v32
!greatern(v30,4) v30 = 4
!greaterv(v30,v32) v30 = v32
!lessn(v30,4) v30 = 4
!lessv(v30,v32) v30 = v32
Also, flags can be tested for by just using the name of the flag:
if (f6) { .....
if (v7 > 0 && !f6) { .....
which is equivalent to:
if (isset(f6)) { .....
if (v7 > 0 && !isset(f6)) { .....
Argument types
There are 9 different types of arguments that commands use:
Type | Prefix |
---|---|
Number | (no prefix) |
v | |
f | |
m | |
o | |
i | |
s | |
w | |
c |
The said test command uses its own special arguments which will be described later.
Each of these types of arguments is given by the prefix and then a number from 0-255, e.g. v5
, f6
, m27
, o2
.
The word type represents words that the player has typed in (as opposed to words that are stored in the WORDS.TOK file). Strings are the temporary string variables stored in memory, not to be confused with messages (that are stored in the logic resources). Controllers are menu items and keys.
Compilers can enforce type checking, so that the programmer must use the correct prefix for an argument so that they know they are using the right type. Decoders should display arguments with the right type.
move.obj(o4, 80, 120, 2, f66);
if (obj.in.box(o2, 30, 60, 120, 40)) { .....
For a complete list of the commands and their argument types, see Logic commands by name.
Messages and inventory items may be given in either numerical or text format:
print("He's not here.");
print(m12);
if (has("Jetpack")) { .....
if (has(i9)) { .....
Messages can also be split over multiple lines:
print("This message is split "
"over multiple lines.");
Quote marks must be used around messages and inventory item names. This is important because some messages or inventory item names may contain parentheses or commas, which could confuse the compiler. This is also the case for the said command which will be described shortly.
if (has("Buckazoid(s)")) { ..... // no ambiguity here about where
// the argument ends
If quote marks are part of the message or inventory object, a \ should be placed in front of these. To use a \, \\ should be used. \n can also be used for a new line.
The said test command uses different parameters to all the other commands. Where as the others use 8 bit arguments (0-255), said takes 16 bit arguments (0-65535). Also, the number of arguments in a said command can vary. The numbers given in the arguments are the word group numbers from the WORDS.TOK file.
if (said(4, 80)) { .....
Words can also be given in place of the numbers:
if (said("look")) { .....
if (said("open","door")) { .....
Quote marks must also be used around the words.
Labels and the goto
command
Labels are given like this:
Label1:
The label name can contain letters, numbers, and the characters '_' and '.'. No spaces are allowed.
The Goto command takes on parameter, the name of a label:
goto(Label1);
Comments
There are three ways that comments can be used.
// - rest of line is ignored
[ - rest of line is ignored
/* Text between these are ignored */
The /*...*/ can be nested:
/* comment start
print("Hello"); // won't be run
/* // a new comment start (will be ignored!)
v32 = 15; // won't be run
*/ // uncomments the most inner comment
print("Hey!"); // won't be run, still inside comments
*/ // uncomments
Note: the fact that these comments can be nested is very different from almost every other language that uses these types of comments, including C, C , and Java.
Defines
See Defines.
Including files
See Includes.
More on messages
In some cases you may want to assign a specific number to a message so you can refer to it in other places. This is done by using the #message command, followed by the number of the message then the message itself:
#message 4 "You can't do that now."
Then you can give the message number as the parameter in commands:
print(m4);
Or embed the message in commands as normal and the number you assigned to it before will be used:
print("You can't do that now.");
#message can be used anywhere in the file, so you do not have to set the message before you use it.
For more details, see Message.
The return
command
The return command is just a normal action command (command number 0), with no arguments. This must be the last command in every logic resource.
See also
Sources
- AGI Studio help file